用DeepSeek模型构建AI 代理
在本综合指南中,我们将探讨如何利用 DeepSeek 的功能构建能够以有意义的方式理解、推理和交互的复杂 AI 代理。
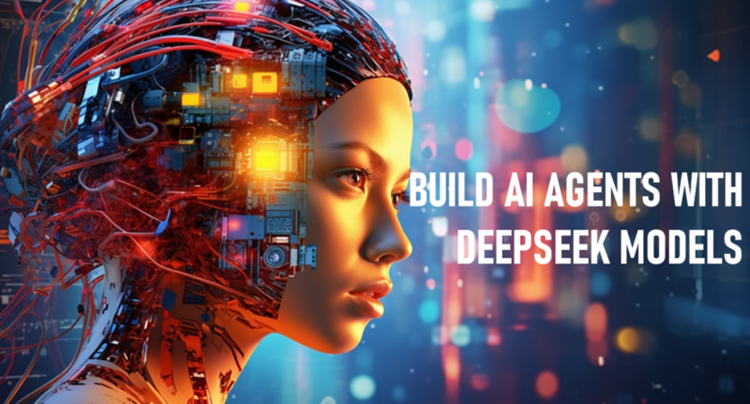
人工智能领域正在迅速发展,DeepSeek 的模型代表了智能 AI 代理开发的重大进步。在本综合指南中,我们将探讨如何利用 DeepSeek 的功能构建能够以有意义的方式理解、推理和交互的复杂 AI 代理。
1、了解 DeepSeek 模型
DeepSeek 推出了一系列强大的语言模型,将强大的推理能力与广泛的知识相结合。这些模型尤其值得注意:
- 高级推理能力,可解决复杂问题
- 强大的编码能力,有助于自动化软件开发
- 强大的自然语言理解能力,可实现类似人类的交互
- 高效的性能,可实现实时应用
2、设置开发环境
在开始构建 AI 代理之前,让我们设置必要的环境。我们将使用 Python 和 DeepSeek API 与模型进行交互。
import os
from deepseek import DeepSeekAPI
# Initialize the DeepSeek client
api_key = os.getenv("DEEPSEEK_API_KEY")
client = DeepSeekAPI(api_key)
# Basic configuration for our agent
class DeepSeekAgent:
def __init__(self, model_name="deepseek-coder-33b-instruct"):
self.client = client
self.model = model_name
self.context = []
def process_input(self, user_input):
# Add input to context
self.context.append({"role": "user", "content": user_input})
# Generate response
response = self.client.generate(
model=self.model,
messages=self.context,
temperature=0.7,
max_tokens=1000
)
# Update context with response
self.context.append({"role": "assistant", "content": response})
return response
3、构建核心代理功能
让我们实现使代理变得智能且有用的基本功能。我们将重点关注三个关键领域:
3.1 自然语言理解
首先,我们将增强代理理解和处理自然语言输入的能力:
class DeepSeekAgent:
def __init__(self, model_name="deepseek-coder-33b-instruct"):
# Previous initialization code...
self.instruction_template = """
Analyze the following input and break it down into:
1. Primary intent
2. Key entities
3. Required actions
Input: {user_input}
"""
def understand_input(self, user_input):
analysis_prompt = self.instruction_template.format(user_input=user_input)
analysis = self.client.generate(
model=self.model,
messages=[{"role": "user", "content": analysis_prompt}],
temperature=0.3
)
return self._parse_analysis(analysis)
def _parse_analysis(self, analysis):
# Implementation of parsing logic
# Returns structured understanding of user input
pass
3.2 推理和决策
接下来,我们将实现代理的推理能力:
def reason_and_plan(self, understanding):
reasoning_prompt = f"""
Based on the following understanding:
{understanding}
Develop a plan of action that:
1. Identifies the optimal approach
2. Considers potential challenges
3. Outlines specific steps to achieve the goal
"""
plan = self.client.generate(
model=self.model,
messages=[{"role": "user", "content": reasoning_prompt}],
temperature=0.4
)
return self._structure_plan(plan)
3.3 操作执行
最后,我们将实现执行层:
def execute_plan(self, plan):
results = []
for step in plan['steps']:
try:
result = self._execute_step(step)
results.append({"step": step, "status": "success", "result": result})
except Exception as e:
results.append({"step": step, "status": "failed", "error": str(e)})
return results
4、高级功能和优化
为了让我们的代理更加复杂,让我们添加一些高级功能:
4.1 内存和上下文管理
class ContextManager:
def __init__(self, max_tokens=4000):
self.memory = []
self.max_tokens = max_tokens
def add_interaction(self, interaction):
self.memory.append(interaction)
self._optimize_memory()
def _optimize_memory(self):
# Implement token counting and context window management
# Remove older interactions when approaching max_tokens
pass
4.2 从互动中学习
我们可以实现一个简单的学习机制:
class LearningModule:
def __init__(self):
self.patterns = {}
self.successful_strategies = {}
def record_interaction(self, interaction, outcome):
# Extract patterns and update successful strategies
pattern = self._extract_pattern(interaction)
if outcome.success:
self._update_strategies(pattern, outcome)
def suggest_strategy(self, current_interaction):
pattern = self._extract_pattern(current_interaction)
return self._find_matching_strategy(pattern)
5、实际应用
让我们看看如何在实际场景中使用我们的代理:
5.1 代码生成助手
def generate_code(self, specification):
prompt = f"""
Create a Python implementation based on this specification:
{specification}
Requirements:
1. Follow PEP 8 style guidelines
2. Include comprehensive documentation
3. Implement error handling
4. Add unit tests
"""
return self.client.generate(
model="deepseek-coder-33b-instruct",
messages=[{"role": "user", "content": prompt}],
temperature=0.2
)
5.2 问题解决代理
def solve_problem(self, problem_description):
steps = [
self.understand_input(problem_description),
self.reason_and_plan,
self.execute_plan
]
solution = self._execute_solution_pipeline(steps)
return self._format_solution(solution)
6、最佳实践和注意事项
使用 DeepSeek 模型构建 AI 代理时,请考虑以下最佳实践:
- 始终实施适当的错误处理和验证
- 监控和优化令牌使用
- 实施速率限制和 API 调用优化
- 定期评估和微调代理性能
- 实施适当的安全措施和输入验证
7、结束语
使用 DeepSeek 模型构建智能 AI 代理为创建复杂的自动化系统开辟了令人兴奋的可能性。通过结合自然语言理解、推理能力和高效执行,我们可以创建在各种应用中提供真正价值的代理。
原文链接:Building Intelligent AI Agents with DeepSeek Models
汇智网翻译整理,转载请标明出处
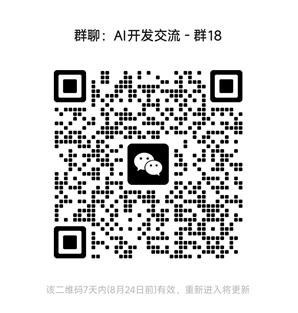