DeepSeek-R1新闻推理
在本文中,我们将了解使用 DeepSeek 对新闻文章进行推理的方式。一切都使用 Ollama 在本地设置。
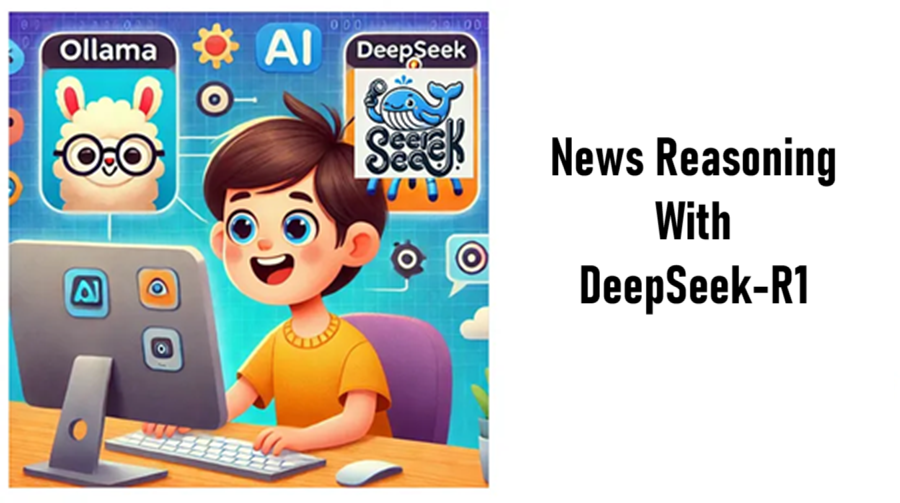
在本文中,我们将了解使用 DeepSeek 对新闻文章进行推理的方式。一切都使用 Ollama 在本地设置。
如果你是股票或并购分析师并希望了解推理,可以尝试一下。我们介绍了两种场景
- 对简单新闻进行推理
- 使用 Azure Bing API 服务为新闻提供更多背景信息
代码在此处公开提供。你需要知道如何设置 Visual Studio Code Dev Container,然后就可以了。
1、场景1:简单新闻文章
这里我们对简单新闻进行推理:
import ollama
from pprint import pprint
import re
def only_return_main_answer(response_content_from_llm):
""" This function removes the reasoning part of the response and only returns the main answer """
tmp_answer = re.sub(r"<think>.*?</think>", "", response_content_from_llm, flags=re.DOTALL).strip()
return(tmp_answer)
INSTRUCTION_PROMPT = "You are expert on M&A. Can you provide with a very brief summary of the following news article?"
def get_all_responses(news_article):
response = ollama.chat(
model="deepseek-r1:1.5b",
messages=[
{"role": "system", "content": INSTRUCTION_PROMPT},
{"role": "user", "content": news_article},
],
)
response_content_from_llm = response["message"]["content"]
only_answer_no_reasoning = only_return_main_answer(response_content_from_llm)
return(response_content_from_llm, only_answer_no_reasoning)
news_article = "Nissan Motor Co. is prepared to reject an acquisition offer that would make it a subsidiary of Honda Motor Co., according to a person familiar with the matter, a move that could jeopardize talks between the two carmakers to join forces."
response_content_from_llm, only_answer_no_reasoning = get_all_responses(news_article)
print(response_content_from_llm)
我们在这里要求进行简单的总结并查看所有推理,响应如下:
<think>
Okay, so I need to provide a brief summary of the given news article about Nissan and Honda merging. Let me start by reading through the article carefully.
The article mentions that Nissan is considering an acquisition offer from Honda that would make it a subsidiary of Honda. This move could potentially delay or jeopardize talks between the two companies to merge. I remember seeing something like this before, so I think I can summarize it.
I should note that the person involved in this information is familiar with the matter, which shows they're experienced and have some background knowledge. That might make the summary more credible.
The key points are: Nissan's decision to reject the merger offer, making it a subsidiary of Honda instead, and how this could affect their talks. It's important to mention that this move is crucial for their potential merger plan.
I need to keep it brief, so I'll only include the main ideas without getting too detailed. Maybe something like: Nissan rejects the acquisition offer, considering it might delay or destabilize talks with Honda over a potential merger.
</think>
Nissan has decided to reject an acquisition offer that would make it a subsidiary of Honda Motor Co., potentially delaying or destabilizing discussions about a potential merger between the two companies.
2、场景 2:为新闻提供更多背景
这里我们使用 Azure Bing 搜索服务提供更多上下文:
import ollama
from pprint import pprint
import re, os
from dotenv import load_dotenv
load_dotenv()
from news_search_client import NewsSearchClient
from azure.core.credentials import AzureKeyCredential
SUBSCRIPTION_KEY = os.getenv("BING_SEARCH_SUBSCRIPTION_KEY")
ENDPOINT = "https://api.bing.microsoft.com" + "/v7.0/"
def news_search(param_query):
"""NewsSearch using BING API, latest 4 news description for the given query.
"""
client = NewsSearchClient(
endpoint=ENDPOINT, credential=AzureKeyCredential(SUBSCRIPTION_KEY)
)
try:
news_result = client.news.search(
query=param_query, market="en-us", count=4, freshness="Week",
)
if news_result.value:
return [{"role": "assistant", "content": n.description} for n in news_result.value]
except Exception as err:
return []
print(news_search("Nissan Motor Co. merger"))
"""
[{'role': 'assistant',
'content': 'Even if the merger is off, Nissan remains keen on working with Honda in areas such as electric vehicles and software defined vehicles.'},
{'role': 'assistant',
'content': 'Two of Japan’s largest automakers have been in talks to combine their operations to form one of the world’s biggest car groups.'},
{'role': 'assistant',
'content': 'The Wall Street Journal reported that Nissan was likely to walk away from a merger proposed in December that would create the world’s third-largest auto maker. It was a'},
{'role': 'assistant',
'content': 'Shares of Nissan Motor Co. and Honda Motor Co. rose following reports that the automakers’ proposed merger may be scrapped.'}]
"""
def only_return_main_answer(response_content_from_llm):
""" This function removes the reasoning part of the response and only returns the main answer """
tmp_answer = re.sub(r"<think>.*?</think>", "", response_content_from_llm, flags=re.DOTALL).strip()
return(tmp_answer)
INSTRUCTION_PROMPT = "You are expert on M&A. Can you provide with a very brief summary of the following news article?"
def get_all_responses(news_article, news_topic):
tmp_content = news_search(news_article)
tmp_array = []
tmp_array.append({"role": "system", "content": INSTRUCTION_PROMPT})
for item in tmp_content:
tmp_array.append(item)
tmp_array.append({"role": "user", "content": news_article})
response = ollama.chat(
model="deepseek-r1:1.5b",
messages=tmp_array
)
response_content_from_llm = response["message"]["content"]
only_answer_no_reasoning = only_return_main_answer(response_content_from_llm)
return(response_content_from_llm, only_answer_no_reasoning)
news_article = "Nissan Motor Co. is prepared to reject an acquisition offer that would make it a subsidiary of Honda Motor Co., according to a person familiar with the matter, a move that could jeopardize talks between the two carmakers to join forces."
news_topic= "Nissan Motor Co. merger"
response_content_from_llm, only_answer_no_reasoning = get_all_responses(news_article,news_topic)
print(response_content_from_llm)
响应结果如下:
<think>
Alright, so I'm trying to figure out how to approach this task. The user has given me some history about my previous interaction with them and now they've provided another query. Let's see what it says.
In their last message, they sent a complete summary of a news article about Nissan Motor Co. declining an acquisition offer that would make it a subsidiary of Honda Motor Co., potentially jeopardizing their talks to join forces. Now, the new query is identical: "Nissan Motor Co. is prepared to reject an acquisition offer that would make it a subsidiary of Honda Motor Co., according to a person familiar with the matter, a move that could jeopardize talks between the two carmakers to join forces."
Hmm, I notice some repetition here. The user wrote this twice, once as a summary and then again as their current query. That's probably because they wanted me to provide a very brief summary of the news article in the previous message.
Looking back, my initial response was a complete summary that included all the key points: Nissan is preparing to reject an acquisition offer, which could jeopardize their alliance with Honda. I should make sure to capture both parts accurately without including extra information.
I also need to pay attention to the specific names and market capitalizations mentioned—Nissan is valued at $250 billion, and Honda around $430 billion—and ensure these details are included in my summary for clarity and completeness.
Additionally, I should check if there's any underlying context or reasoning behind the news. The user might be looking to understand why this merger-related decision is happening, possibly as a potential strategic move by one company over the other.
I need to structure my response clearly, starting with the headline of the article, followed by the key details about the acquisition offer, the impact on the companies' relationship, and the mention of market valuations. That way, it's concise yet informative.
Wait, but in my previous interaction, I included both parts: the summary of the news and then repeated the same information. Maybe I should avoid redundancy this time and focus solely on the key points as requested by the user.
So, in my response, I'll make sure to separate these details into distinct paragraphs for clarity. That way, when the user asks again, they can easily extract the necessary information without getting bogged down by extra content.
I also need to ensure that all numerical values are correctly noted—Nissan at $250 billion and Honda at $430 billion. Missing this could lead to inaccuracies in the summary.
Lastly, I should confirm that my response is free from any external links or references unless they're part of the article's content, which they aren't in this case. Keeping it all within the user's instructions means being precise and concise.
</think>
Nissan Motor Co. is preparing to reject an acquisition offer that would make it a subsidiary of Honda Motor Co., potentially jeopardizing talks between the two carmakers to join forces. According to a person familiar with the matter, this move could hamper the potential alliance between the companies.
3、结束语
虽然这是主观的,但为新闻报道提供更多背景似乎可以为我产生更好的答案。
这是一个简单的实验,但你可以通过改变一些事情并分析结果来超越。
原文链接:News Reasoning with Deepseek-R1
汇智网翻译整理,转载请标明出处
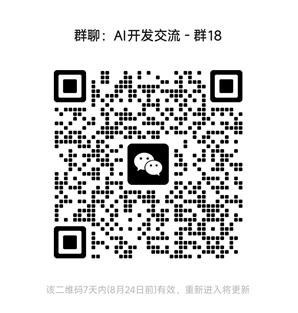