Vanna:SQL生成专用RAG框架
Vanna 是一个 MIT 许可的开源 Python RAG框架,用于 SQL 生成和相关功能。
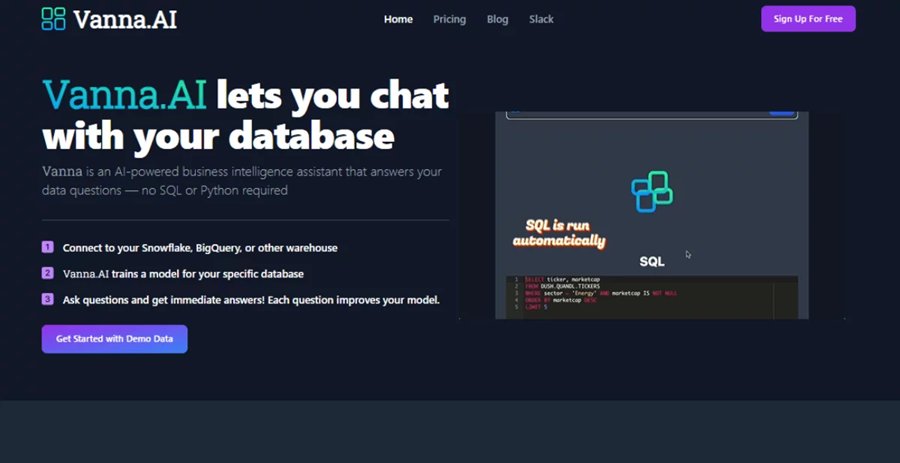
Vanna 是一个 MIT 许可的开源 Python RAG(检索增强生成)框架,用于 SQL 生成和相关功能。
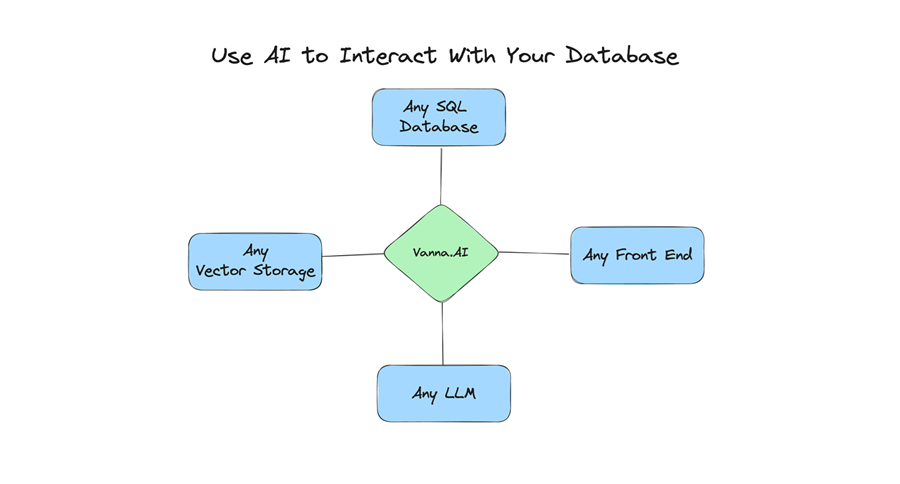
Vanna支持的大模型包括:OpenAI、Anthropic、Gemini、Ollama、Qianwen、AWS Bedrock、Huggingface、Qianfan等。
Vanna支持的向量数据库包括:PgVector、PineCore、ChromaDB、Milvus、Qdrant、Weaviate、Margo、FAISS等
Vanna支持的SQL数据库包括:PostgreSQL、MySQL、PrestoDB、Apache Hive、Oracle、Snowflake、MS SQL Server、BigQuery、SQLite、DuckDB等。
下面这些是我们使用 Vanna 构建的一些用户界面。你可以按原样使用这些界面,也可以将其作为你自己的自定义界面的起点:笔记本、Streamlit界面、Flask界面、Slack界面。
1、Vanna 的工作原理
从本质上讲,Vanna 是一个 Python 包,它使用检索增强功能来帮助你使用 LLM 为数据库生成准确的 SQL 查询。
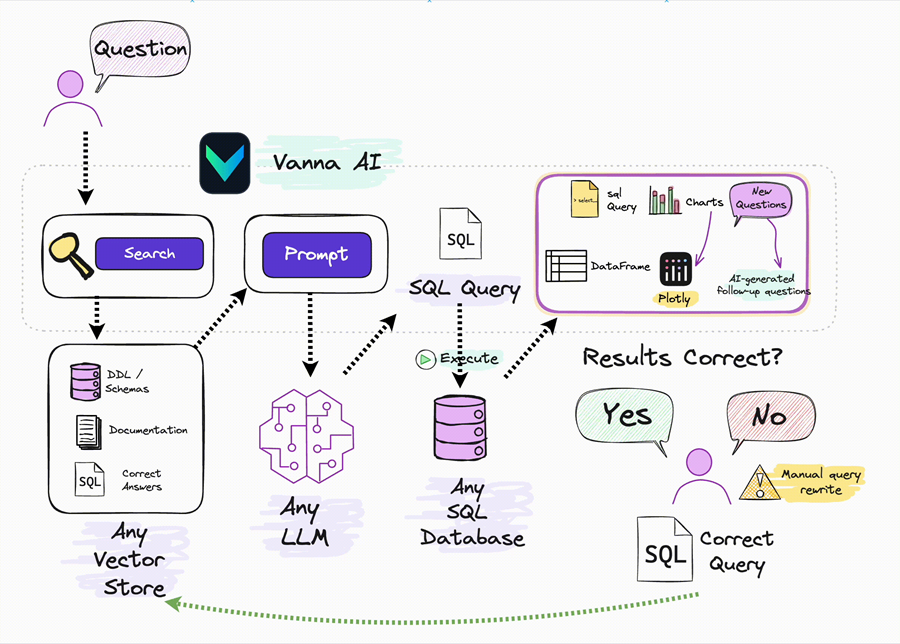
Vanna 的工作分为两个简单的步骤 - 在你的数据上训练 RAG“模型”,然后提出问题,这些问题将返回可设置为在你的数据库上自动运行的 SQL 查询。
vn.train(...)
在你的数据上训练 RAG“模型”。这些方法添加到下面的参考语料库中。
vn.ask(...)
提出问题。这将使用参考语料库生成可以在你的数据库上运行的 SQL 查询。
2、使用示例数据快速入门
!pip install vanna
import vanna
from vanna.remote import VannaDefault
vn = VannaDefault(model='chinook', api_key=vanna.get_api_key('my-email@example.com'))
vn.connect_to_sqlite('https://vanna.ai/Chinook.sqlite')
vn.ask("What are the top 10 albums by sales?")
from vanna.flask import VannaFlaskApp
VannaFlaskApp(vn).run()
运行结果示例如下:
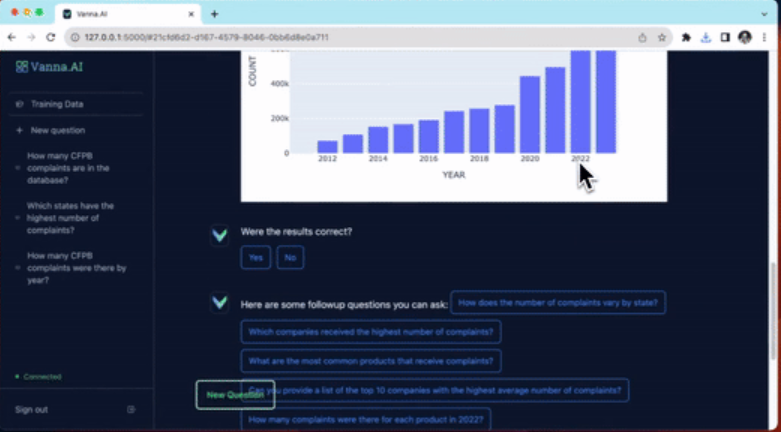
3、使用 OpenAI 为 Postgres 生成 SQL
这个笔记本介绍了使用 vanna Python 包通过 AI(RAG + LLM)生成 SQL 的过程,包括连接到数据库和进行训练。如果你尚未准备好在自己的数据库上进行训练,仍然可以使用示例 SQLite 数据库进行尝试。
3.1 环境设置
%pip install 'vanna[postgres]'
import vanna
from vanna.remote import VannaDefault
api_key = # Your API key from https://vanna.ai/account/profile
vanna_model_name = # Your model name from https://vanna.ai/account/profile
vn = VannaDefault(model=vanna_model_name, api_key=api_key)
连接Postgres数据库:
vn.connect_to_postgres(host='my-host', dbname='my-dbname', user='my-user', password='my-password', port='my-port')
3.2 训练
你只需训练一次。除非你想添加更多训练数据,否则无需再次训练:
# The information schema query may need some tweaking depending on your database. This is a good starting point.
df_information_schema = vn.run_sql("SELECT * FROM INFORMATION_SCHEMA.COLUMNS")
# This will break up the information schema into bite-sized chunks that can be referenced by the LLM
plan = vn.get_training_plan_generic(df_information_schema)
plan
# If you like the plan, then uncomment this and run it to train
# vn.train(plan=plan)
# The following are methods for adding training data. Make sure you modify the examples to match your database.
# DDL statements are powerful because they specify table names, colume names, types, and potentially relationships
vn.train(ddl="""
CREATE TABLE IF NOT EXISTS my-table (
id INT PRIMARY KEY,
name VARCHAR(100),
age INT
)
""")
# Sometimes you may want to add documentation about your business terminology or definitions.
vn.train(documentation="Our business defines OTIF score as the percentage of orders that are delivered on time and in full")
# You can also add SQL queries to your training data. This is useful if you have some queries already laying around. You can just copy and paste those from your editor to begin generating new SQL.
vn.train(sql="SELECT * FROM my-table WHERE name = 'John Doe'")
# At any time you can inspect what training data the package is able to reference
training_data = vn.get_training_data()
training_data
# You can remove training data if there's obsolete/incorrect information.
vn.remove_training_data(id='1-ddl')
```## Asking the AI
Whenever you ask a new question, it will find the 10 most relevant pieces of training data and use it as part of the LLM prompt to generate the SQL.
```python
vn.ask(question=...)
3.3 启动用户界面
from vanna.flask import VannaFlaskApp
app = VannaFlaskApp(vn)
app.run()
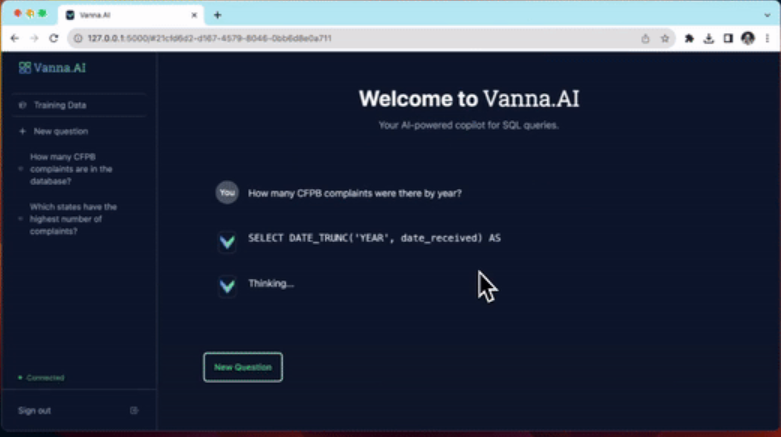
4、Vanna训练函数
vn.train
是一个包装函数,允许你训练系统(即位于 LLM 之上的检索增强层)。可以通过以下方式调用它:
4.1 DDL 语句
这些语句让系统了解可用的表、列和数据类型:
vn.train(ddl="CREATE TABLE my_table (id INT, name TEXT)")
4.2 文档字符串
这些可以是任何有关你的数据库、业务或行业的文档,这些文档可能是 LLM 了解用户问题背景所必需的:
vn.train(documentation="Our business defines XYZ as ABC")
4.3 SQL 语句
对系统来说,最有帮助的事情之一是你的组织中常用的 SQL 查询。这将有助于系统了解所提问题的上下文:
vn.train(sql="SELECT col1, col2, col3 FROM my_table")
4.4 问题-SQL 对
你还可以使用问题-SQL 对来训练系统。这是训练系统的最直接方法,也是帮助系统理解所提问题上下文的最有效方法:
vn.train(
question="What is the average age of our customers?",
sql="SELECT AVG(age) FROM customers"
)
问题-SQL 对包含大量嵌入信息,系统可以使用这些信息来理解问题的上下文。当你的用户倾向于提出具有很多歧义的问题时,尤其如此。
4.5 训练计划
# The information schema query may need some tweaking depending on your database. This is a good starting point.
df_information_schema = vn.run_sql("SELECT * FROM INFORMATION_SCHEMA.COLUMNS")
# This will break up the information schema into bite-sized chunks that can be referenced by the LLM
plan = vn.get_training_plan_generic(df_information_schema)
plan
# If you like the plan, then uncomment this and run it to train
vn.train(plan=plan)
训练计划基本上就是将你的数据库信息模式分解为 LLM 可以引用的小块。这是一种快速使用大量数据训练系统的好方法。
原文连接:Vanna - RAG framework for SQL generation
汇智网翻译整理,转载请标明出处